AppHarbor is running a contest with Twilio and to get you started, we have asked Twilio developer and all-round great-guy John Sheehan to write a guest post on how to integrate voice and sms in your .NET apps.
My name is John Sheehan and I'm a .NET developer working for Twilio. Twilio is the easiest way to send and receive phone calls from your applications. One of our most common use cases is handling incoming calls. To accomplish this, you provide a XML document on a public URL that Twilio accesses when one of your phone numbers receives a call. Using simple markup commands called TwiML, you can instruct Twilio to speak text to the caller, gather input via touchtones, record audio from the caller, dial other numbers and much more.
Until recently, .NET developers looking to get started using Twilio had a big barrier to overcome to make TwiML scripts publicly available for use with our system. Existing hosting options are overly-complicated and costly to set up and maintain. Deployment is also a challenge with FTP or WebDeploy being the most frequently available options. AppHarbor changes everything for Twilio .NET developers. As of today, AppHarbor is the fastest and easiest way to get your Twilio voice and SMS applications up and running.
We're so excited about what AppHarbor is doing that we've partnered with them for this week's Twilio developer contest. The best Twilio voice or SMS app running on AppHarbor that is submitted by this Sunday at 11:59pm PT will win a Samsung Focus Windows Phone 7 ($500 value), $500 in AppHarbor credit and $100 in Twilio credit. You can read more about the contest on the Twilio blog.
To help get you started writing your first Twilio app, here are a couple examples of using our API in ASP.NET MVC and WebMatrix.
ASP.NET MVC
Returning TwiML from an ASP.NET MVC controller action is a straightforward process. There are a couple key considerations:
- Set the response content type to text/xml
- Generate valid TwiML XML
- Return generated TwiML, no views needed
Let's take a look at a sample controller action. We'll write a simple voicemail system that prompts the caller to leave a message and then records the caller's message.
public ActionResult HandleCall(string To, string From, string CallSid)
{
var doc = new XDocument();
var response = new XElement("Response");
response.Add(new XElement("Say", "Thank you for calling, please leave a message."));
response.Add(new XElement("Record",
new XAttribute("maxLength", 30),
new XAttribute("action", Url.Action("HandleRecording"))
));
doc.Add(response);
return new ContentResult
{
ContentType = "text/xml",
Content = doc.ToString()
};
}
While a lot of this could be abstracted to be easier it shows the general concepts involved with generating TwiML. When the caller completes the recording a POST request will be made to /HandleRecording with the data for you to process. More info on that process can be found here.
To get this up and running you would push your site to AppHarbor, then assign the URL to one of your Twilio numbers. When a call arrives to your number, a POST request is made to your URL and the call starts.
WebMatrix and Razor (ASP.NET Web Pages)
Because Razor is such a great templating language for generating bracketed syntax, it works great with TwiML. Replicating the same functionality from above gets us the following code. Drop this into a .cshtml file, push to AppHarbor and you're ready to go.
@{
Response.ContentType = "text/xml";
var to = Request["To"];
var from = Request["From"];
var callSid = Request["CallSid"]; // unique call ID common across requests for this call
}
<Response>
<Say>Thank you for calling, please leave a message.</Say>
<Record action="HandleRecording" maxLength="30" />
</Response>
In both examples I grab some of the common data sent with every request from Twilio to your servers. You can use this info to dynamically control the call, keep detailed logs and more.
This just barely scratches the surface of what you can do using Twilio. We also offer APIs for sending and receiving SMS. You can also initiate outbound calls via the REST API and control them with TwiML just like an incoming call. And best of all, AppHarbor provides a ridiculously great platform for running Twilio apps. Use your creativity to build the best Twilio app running on AppHarbor and win yourself a Windows Phone 7!
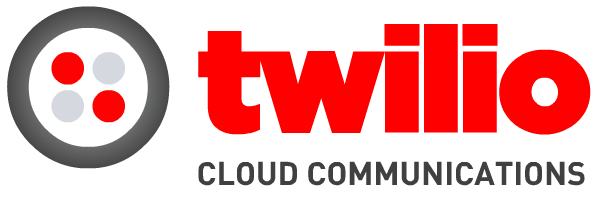